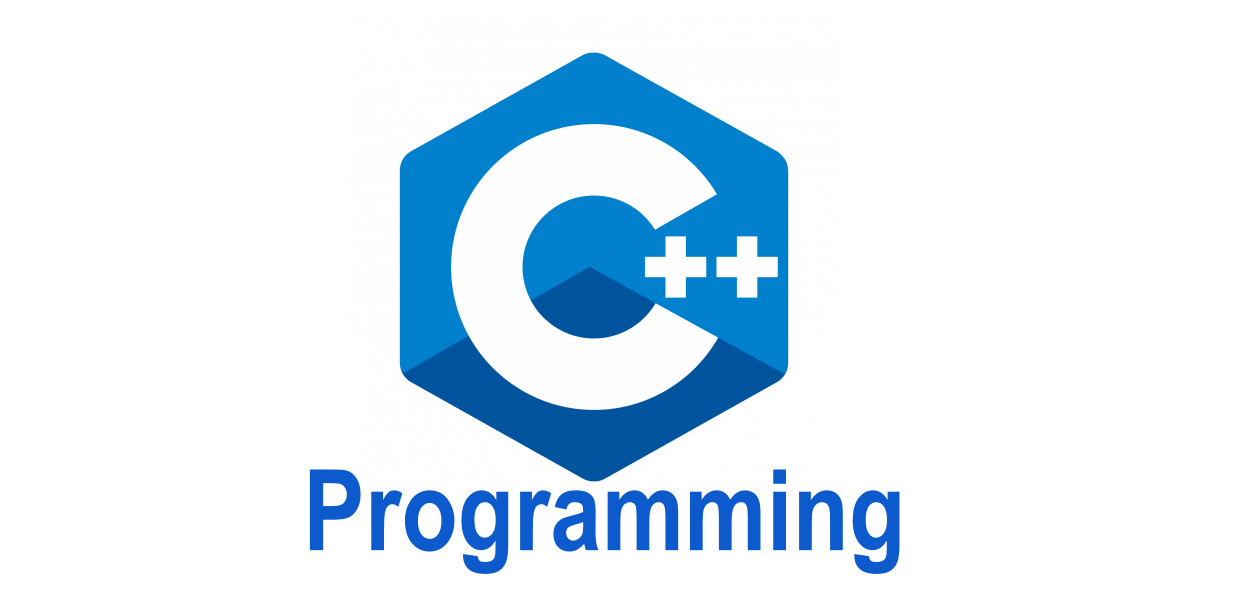
Introduction to C++
- Creating a project
- Writing, compiling and running a program
Variables and data types
- Expressions
- Constants
- Operators
- Type conversions
Looping constructs: while, do…while, for loops
- If…else statements
- Switch/case construct
Functions
- Passing arguments
- Function prototyping
- Default argument initializers
- Inline functions
Arrays
- Array initialisation
- Multi-dimensional arrays
- Character arrays
- Working with character strings
Storage Classes
- Global variables
Pointers
- Pointer and arrays
- Pointers to character strings
- Arrays of pointers
- Memory slicing
- Pointers to functions
C++ classes
- Data members and member functions
- Creating objects
- The new and delete operators
- Friends to a class
- Class initialisation
Reference types
- Reference type arguments
Function overloading
- Operator overloading
Copy constructor
- Assignment operator
Template classes
- Static class members
- File streams
Inheritance
- Base classes and derived classes
- Inherited member access
- Base class initialisation
- Protected members of a class
Virtual functions
- Virtual destructors
Virtual base classes
- Virtual base class member access
- Constructor and destructor ordering
Exception handling
- try…throw…catch block
- Nested catch handlers